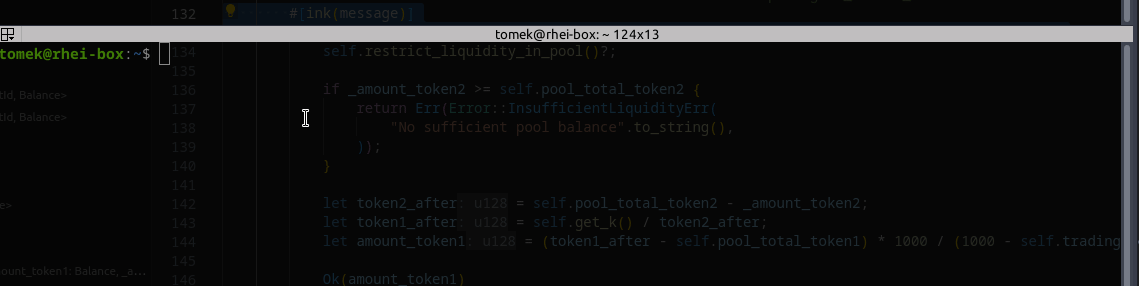
JavaScript
function in object
1const obj = {2 value: 'some value',3 method() { return this.value; }4};5obj.method(); // "some value"67const x = obj.method;8x(); // undefined
TypeScript
1tsc --watch //watch mode
Logical nullish assignment
1function myFn(variable1, variable2) {2 variable2 ??= "default value"3 return variable1 + variable24}56myFn("this has", " no default value") //returns "this has no default value"7myFn("this has no") //returns "this has no default value"8myFn("this has no", 0) //returns "this has no 0"
The assignment operator allows us to check for the value of variable2 and if it evaluates to either null
or undefined
then the assignment will go through, otherwise it will never happen.
Warning: this syntax can be confusing to others that aren’t familiar with this operator, so if you’re using it you might as well leave a one-line comment explaining what’s happening.
Observables
TL;DR: You want a HOT observable when you don’t want to create your producer over and over again.
1// COLD2var cold = new Observable((observer) => {3 var producer = new Producer();4 // have observer listen to producer here5});
HOT is when your observable closes over the producer
1// HOT2var producer = new Producer();3var hot = new Observable((observer) => {4 // have observer listen to producer here5});
Comparing promise’s then
to observable’s subscribe
, showing differences in eager vs lazy execution, showing cancellation and reuse of observables, etc. It’s a handy way to introduce beginners to observables.
There’s one problem: Observables are more different from promises than they are similar. Promises are always multicast. Promise resolution and rejection is always async. When people approach observables as though they’re similar to a promises, they expect these things, but they’re not always true. Observables are sometimes multicast. Observables are usually async. I blame myself a little, I’ve helped perpetuate this misunderstanding.
Observable is just a function that takes an observer and returns a function
If you really want to understand observable, you could simply write one. It’s not as hard as it sounds, honestly. An observable, boiled down to it’s smallest parts, is no more than a specific type of function with a specific purpose.
Shape
- A function that accepts an observer: An object with
next
,error
andcomplete
methods on it. - And returns a cancellation function
Purpose
To connect the observer to something that produces values (a producer), and return a means to tear down that connection to the producer. The observer is really a registry of handlers that can be pushed values over time.
1// simple implementation of observable2function myObservable(observer) {3 const datasource = new DataSource();4 datasource.ondata = (e) => observer.next(e);5 datasource.onerror = (err) => observer.error(err);6 datasource.oncomplete = () => observer.complete();7 return () => {8 datasource.destroy();9 };10}
Observable
An Observable is a data stream that houses data that can be passed through different threads. In our demo app, we’ll be using an Observable to supply data to our different components.
Observer
An Observer consumes the data supplied by an Observable. In our demo app, we’ll be using our setState
Hook to consume data from our Observable.
Subscription
In order for our Observer to consume data from our Observable, we’ll have to subscribe it to the Observable. In our demo app, we’ll be using the subscribe()
method to subscribe our setState
Observer to our Observable.
switchMap
cancels previous HTTP requests that are still in progress, while mergeMap
lets all of them finish
So here’s the simple difference — switchMap
cancels previous HTTP requests that are still in progress, while mergeMap
lets all of them finish.
In my case, I needed all requests to go through, as this is a metrics service that’s supposed to log all actions that the user performs on the web page, so I used mergeMap.
A good use case for switchMap
would be an autocomplete input, where we should discard all but the latest results from the user’s input.
Rust
Example of passing arguments by reference in Rust:
1fn main() {2 // Define a mutable variable and a reference to it3 let mut n_main: usize = 100;4 let n_main_ref: &usize = &n_main;56 // Prints the return value of `increment_value`, original variable unchanged7 println!("{}", increment_value(n_main_ref)); // "101"8 println!("n_main = {}", n_main); // "n_main = 100"910 // This function receives a mutable reference, and changes the original value.11 amend(&mut n_main);12 println!("n_main = {}", n_main); // "n_main = 199"13}1415// Accepts a mutable reference, changes the value it refers to, no return value16fn amend(n_inc_ref: &mut usize) {17 *n_inc_ref +=99;18}1920// Accepts a reference, does not mutate it, returns same type as the passed-in reference21fn increment_value(n_inc_ref: &usize) -> usize {22 n_inc_ref + 123}
1.unwrap //should be applied only on dev environments
https://github.com/holmgr/cargo-sweep
WebAssembly
Tokio works with WASM when:
1tokio = { version = "1.28.2", features = ["macros", "sync"] }
Python
1str != repr
OCaml
http://wazniak.mimuw.edu.pl/index.php?title=Programowanie_funkcyjne/Wst%C4%99p
Substrate
1#ifwedontbuildittheycantuseit
https://brson.github.io/2020/12/03/substrate-and-ink-part-1
Solidity
tbd
Wasm
tbd
React
tbd
Algorithms
Ligo
CSS
Coffee time
Dear Reader, if you think the article is valuable to you and you want me to drink (and keep writing) high quality coffee (I do like it), feel free to buy me a cup of coffee. ⚡